Even or Odd in JavaScript (Solved)
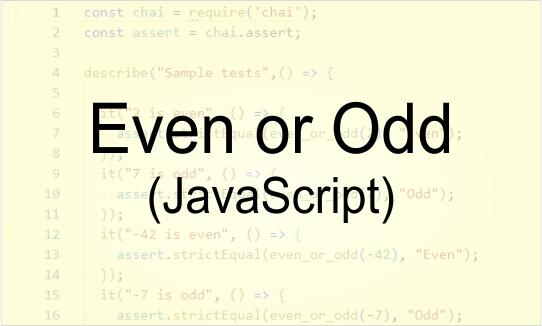
Create a function that takes an integer as an argument and returns "Even" for even numbers or "Odd" for odd numbers.
Solution
function even_or_odd(number) {
return number % 2 ? "Odd" : "Even";
}
Even Numbers
Any integer that can be divided exactly by 2 is an even number.
The last digit is 0, 2, 4, 6 or 8
Example: −24, 0, 6 and 38 are all even numbers
Odd Numbers
Any integer that cannot be divided exactly by 2 is an odd number.
The last digit is 1, 3, 5, 7 or 9
Example: −3, 1, 7 and 35 are all odd numbers
Odd numbers are in between the even numbers.
Integers
Integers are like whole numbers, but they also include negative numbers ... but still no fractions allowed!
So, integers can be negative {−1, −2,−3, −4, ... }, positive {1, 2, 3, 4, ... }, or zero {0}
We can put that all together like this:
Integers = { ..., −4, −3, −2, −1, 0, 1, 2, 3, 4, ... }
Examples: −16, −3, 0, 1 and 198 are all integers. (But numbers like ½, 1.1 and 3.5 are not integers)
Conditional (ternary) operator
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy
followed by a colon (:), and finally the expression to execute if the condition is falsy
. This operator is frequently used as an alternative to an if...else
statement.
Syntax
condition ? exprIfTrue : exprIfFalse
Parameters
- condition
-
An expression whose value is used as a condition.
- exprIfTrue
-
An expression which is executed if the condition evaluates to a
truthy
value (one which equals or can be converted to true). - exprIfFalse
-
An expression which is executed if the condition is
falsy
(that is, has a value which can be converted to false).
Description
Besides false, possible falsy expressions are: null, NaN, 0, the empty string (""), and undefined. If condition is any of these, the result of the conditional expression will be the result of executing the expression exprIfFalse.
Cracking the Coding Interview: 189 Programming Questions and Solutions
Cracking the Coding Interview: 189 Programming Questions and Solutions 6th Edition by Gayle Laakmann McDowell I am not a recruiter. I am a software engineer. And as such, I know what it's like to be asked to whip up brilliant algorithms on the spot and then write flawless code on a whiteboard. I've been through this as a candidate and as an interviewer. Cracking the Coding Interview, 6th Edition is here to help you through this process, teaching you what you need to know and enabling you to perform at your very best. I've coached and interviewed hundreds of software engineers. The result is this book. Learn how to uncover the hints and hidden details in a question, discover how to break down a problem into manageable chunks, develop techniques to unstick yourself when stuck, learn (or re-learn) core computer science concepts, and practice on 189 interview questions and solutions. These interview questions are real; they are not pulled out of computer science textbook…
The Self-Taught Programmer: The Definitive Guide by Cory Althoff
The Self-Taught Programmer: The Definitive Guide to Programming Professionally by Cory Althoff I am a self-taught programmer. After a year of self-study, I learned to program well enough to land a job as a software engineer II at eBay. Once I got there, I realized I was severely under-prepared. I was overwhelmed by the amount of things I needed to know but hadn't learned yet. My journey learning to program, and my experience at my first job as a software engineer were the inspiration for this book. This book is not just about learning to program; although you will learn to code. If you want to program professionally, it is not enough…
Hands-On Machine Learning 2nd Edition by Aurélien Géron
Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow: Concepts, Tools, and Techniques to Build Intelligent Systems 2nd Edition by Aurélien Géron Through a series of recent breakthroughs, deep learning has boosted the entire field of machine learning. Now, even programmers who know close to nothing about this technology can use simple, efficient tools to implement programs capable of learning from data. This practical book shows you how. By using concrete…