Multiply in JavaScript (Solved)
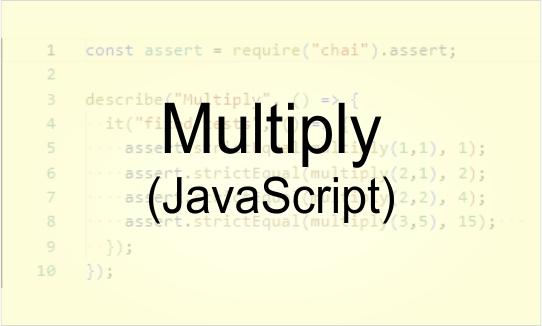
This code does not execute properly. Try to figure out why.
Solution
function multiply(a, b) {
return a * b;
}
Multiplication
The multiplication of whole numbers may be thought of as repeated addition; that is, the multiplication of two numbers is equivalent to adding as many copies of one of them, the multiplicand, as the quantity of the other one, the multiplier. Both numbers can be referred to as factors.
Example: 3 * 4 = 4 + 4 + 4 = 12
return
The return statement ends function execution and specifies a value to be returned to the function caller.
Syntax
return [expression];
Parameters
- expression
-
The expression whose value is to be returned. If omitted, undefined is returned instead.
Description
When a return statement is used in a function body, the execution of the function is stopped. If specified, a given value is returned to the function caller.
How to detect which one of the defined font was used in a web page?
Suppose I have the following CSS rule in my page: body { font-family: Calibri, Trebuchet MS, Helvetica, sans-serif; } How could I detect which one of the defined fonts were used in the user's browser? For people wondering why I want to do this is because the font I'm detecting contains glyphs that are not available in other fonts. If the user does not have the font, then I want it to display a link asking the user to download that font (so they can use my web application with the correct font). Currently, I am displaying the download font link for all users. I want to only display this for people who do not have the correct font installed. One thing to keep in mind is that some browsers will replace certain missing fonts with similar fonts, which is impossible to detect using the JavaScript/CSS trick. For example, Windows browsers will substitute Arial for Helvetica if it's not installed. The…
Even or Odd | JavaScript (solved) | codewars
Even or Odd in JavaScript (Solved) Create a function that takes an integer as an argument and returns "Even" for even numbers or "Odd" for odd numbers. Solution function even_or_odd(number) { return number % 2 ? "Odd" : "Even"; } Even Numbers Any integer that can be divided exactly by 2 is an even number . The last digit is 0, 2, 4, 6 or 8 Example: −24, 0, 6 and 38 are all even numbers Odd Numbers Any integer that cannot be divided exactly by 2 is an odd number . The last digit is 1, 3, 5, 7 or 9 Example: −3, 1, 7 and 35 are all odd numbers Odd numbers are in between the even numbers. Integers Integers are like whole numbers, but they also include…
Cracking the Coding Interview: 189 Programming Questions and Solutions
Cracking the Coding Interview: 189 Programming Questions and Solutions 6th Edition by Gayle Laakmann McDowell I am not a recruiter. I am a software engineer. And as such, I know what it's like to be asked to whip up brilliant algorithms on the spot and then write flawless code on a whiteboard. I've been through this as a candidate and as an interviewer. Cracking the Coding Interview, 6th Edition is here to help you through this process, teaching you what you need to know…