Triple Quotes? How do I delimit a databound Javascript string parameter in ASP . NET?
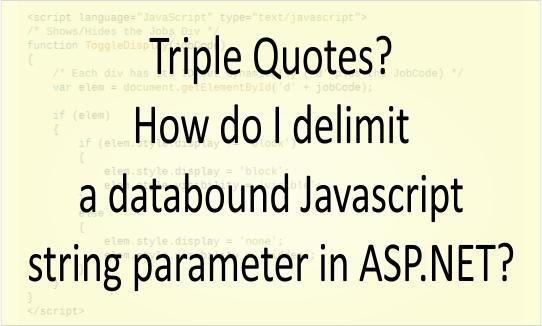
How do I delimit a Javascript data-bound string parameter in an anchor OnClick
event?
- I have an anchor tag in an ASP . NET Repeater control.
- The
OnClick
event of the anchor contains a call to a Javascript function. - The Javascript function takes a string for its input parameter.
- The string parameter is populated with a data-bound value from the Repeater.
I need the "double quotes" for the Container.DataItem
.
I need the 'single quotes' for the OnClick
.
And I still need one more delimiter (triple quotes?) for the input string parameter of the Javascript function call.
Since I can't use 'single quotes' again, how do I ensure the Javascript function knows the input parameter is a string and not an integer?
Without the extra quotes around the input string parameter, the Javascript function thinks I'm passing in an integer.
The anchor:
<a id="aShowHide" onclick='ToggleDisplay(<%# DataBinder.Eval(Container.DataItem, "JobCode") %>);' >Show/Hide</a>
And there is my Javascript:
<script language="JavaScript" type="text/javascript">
/* Shows/Hides the Jobs Div */
function ToggleDisplay(jobCode)
{
/* Each div has its ID set dynamically ('d' plus the JobCode) */
var elem = document.getElementById('d' + jobCode);
if (elem)
{
if (elem.style.display != 'block')
{
elem.style.display = 'block';
elem.style.visibility = 'visible';
}
else
{
elem.style.display = 'none';
elem.style.visibility = 'hidden';
}
}
}
</script>
5 Answers
I had recently similar problem and the only way to solve it was to use plain old HTML codes for single (') and double quotes (").
Source code was total mess of course but it worked.
Try
<a id="aShowHide" onclick='ToggleDisplay("<%# DataBinder.Eval(Container.DataItem, "JobCode") %>");'>Show/Hide</a>
or
<a id="aShowHide" onclick='ToggleDisplay('<%# DataBinder.Eval(Container.DataItem, "JobCode") %>');'>Show/Hide</a>
Winna!!! Thanks Lubos. HTML codes sorted it (and yep, it was messy, but it works). Solution: onclick='ToggleDisplay("<%# DataBinder.Eval(Container.DataItem, "JOB_Code") %>");'
" is double quote. ' is single quote
onclick='javascript:ToggleDisplay("<%# DataBinder.Eval(Container.DataItem, "JobCode")%> "); '
Use like above.
Without the extra quotes around the input string parameter, the Javascript function thinks I'm passing in an integer.
Can you do some rudimentary string function to force JavaScript into changing it into a string? Like
value = value + ""
Try putting the extra text inside the server-side script block and concatenating.
onclick='<%# "ToggleDisplay(""" & DataBinder.Eval(Container.DataItem, "JobCode") & """);" %>'
Edit: I'm pretty sure you could just use double quotes outside the script block as well.
Passing variable to function without single quote or double quote
<html>
<head>
</head>
<body>
<script language="javascript">
function hello(id, bu)
{
alert(id+ bu);
}
</script>
<a href ="javascript:
var x = "12";
var y = "fmo";
hello(x,y)">test</a>
</body>
</html>
Document.getElementById()
An Element object describing the DOM element object matching the specified ID, or null if no matching element was found in the document.
Syntax
getElementById(id)
Parameters
- id
-
The ID of the element to locate. The ID is case-sensitive string which is unique within the document; only one element may have any given ID.
Description
The Document method getElementById() returns an Element object representing the element whose id property matches the specified string. Since element IDs are required to be unique if specified, they're a useful way to get access to a specific element quickly.
If you need to get access to an element which doesn't have an ID, you can use querySelector() to find the element using any selector.
DataBinder.Eval Method
Uses reflection to parse and evaluate a data-binding expression against an object at run time.
Overloads
- Eval(Object, String)
-
Evaluates data-binding expressions at run time.
- Eval(Object, String, String)
-
Evaluates data-binding expressions at run time and formats the result as a string.
Syntax (Eval(Object, String))
Evaluates data-binding expressions at run time.
C#
public static object Eval (object container, string expression);
Parameters
- container
-
Object. The object reference against which the expression is evaluated. This must be a valid object identifier in the page's specified language.
- expression
-
String. The navigation path from the container object to the public property value to be placed in the bound control property. This must be a string of property or field names separated by periods, such as Tables[0].DefaultView.[0].Price in C# or Tables(0).DefaultView.(0).Price in Visual Basic.
Description
The value of the expression parameter must evaluate to a public property.
This method is automatically called when you create data bindings in a rapid application development (RAD) designer such as Visual Studio. You can also use it declaratively to simplify casting to a text string. To do so, you use the <%# %> expression syntax, as used in standard ASP . NET data binding.
This method is particularly useful when binding data to controls that are in a templated list.
Eval(Object, String, String)
Evaluates data-binding expressions at run time and formats the result as a string.
C#
public static string Eval (object container, string expression, string format);
Parameters
- container
-
Object. The object reference against which the expression is evaluated. This must be a valid object identifier in the page's specified language.
- expression
-
String. The navigation path from the container object to the public property value to be placed in the bound control property. This must be a string of property or field names separated by periods, such as Tables[0].DefaultView.[0].Price in C# or Tables(0).DefaultView.(0).Price in Visual Basic.
- format
-
String. A .NET Framework format string (like those used by Format(String, Object)) that converts the Object instance returned by the data-binding expression to a String object.
Description
The value of expression must evaluate to a public property.
For more information about format strings in the .NET Framework, see Formatting Types.
This method is automatically called when you create data bindings in a rapid application development (RAD) designer such as Visual Studio. You can also use it declaratively to convert the Object resulting from the data-binding expression to a String. To use the method declaratively, use the <%# %> expression syntax, as used in standard ASP . NET data binding.
This method is particularly useful when binding data to controls that are in a templated list.
ASP . NET Custom Client-Side Validation
I have a custom validation function in JavaScript in a user control on a .Net 2.0 web site which checks to see that the fee paid is not in excess of the fee amount due. I've placed the validator code in the ascx file, and I have also tried using Page.ClientScript.RegisterClientScriptBlock() and in both cases the validation fires, but cannot find the JavaScript function. The output in Firefox's error console is "feeAmountCheck is not defined" . Here is the function (this was taken directly from firefox->view source) <script type="text/javascript"> function feeAmountCheck(source, arguments) { var amountDue = document.getElementById('ctl00_footerContentHolder_Fees1_FeeDue'); var amountPaid = document.getElementById('ctl00_footerContentHolder_Fees1_FeePaid'); if (amountDue.value > 0 && amountDue >= amountPaid) { arguments.Is…
Multiply | JavaScript (solved) | codewars
This code does not execute properly. Try to figure out why. Solution function multiply(a, b) { return a * b; } Multiplication The multiplication of whole numbers may be thought of as repeated addition; that is, the multiplication of two numbers is equivalent to adding as many copies of one of them, the multiplicand, as the quantity of the other one, the multiplier. Both numbers can be referred to as factors. Example: 3 * 4 = 4 + 4 + 4 = 12 return The return statement ends function execution and specifies a value to be returned to the function caller. Syntax return [expression]; Parameters expression The expression whose value…
How to detect which one of the defined font was used in a web page?
Suppose I have the following CSS rule in my page: body { font-family: Calibri, Trebuchet MS, Helvetica, sans-serif; } How could I detect which one of the defined fonts were used in the user's browser? For people wondering why I want to do this is because the font I'm detecting contains glyphs that are not available in other fonts. If the user does not have the font, then I want it to display a link asking the user to download that font (so they can use my web application…