How to specify javascript to run when ModalPopupExtender is shown
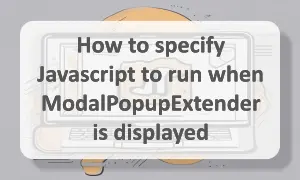
The ASP _ NET AJAX ModalPopupExtender
has OnCancelScript
and OnOkScript
properties, but it doesn't seem to have an OnShowScript
property. I'd like to specify a javascript function to run each time the popup is shown.
In past situations, I set the TargetControlID
to a dummy control and provide my own control that first does some JS code and then uses the JS methods to show the popup. But in this case, I am showing the popup from both client and server side code.
Anyone know of a way to do this?
BTW, I needed this because I have a textbox in the modal that I want to make a TinyMCE editor. But the TinyMCE init script doesn't work on invisible textboxes, so I had to find a way to run it at the time the modal was shown.
They show you how to RAISE an event manually, not not how to attach to it: asp _ net _ ajaxlibrary _ act_modalpopup.ashx ...priceless
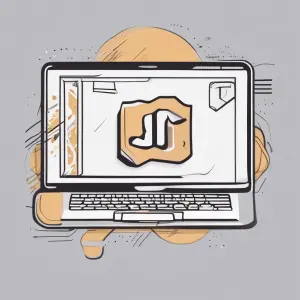
8 Answers
hmmm... I'm pretty sure that there's a shown event for the MPE... this is off the top of my head, but I think you can add an event handler to the shown event on page_load
function pageLoad()
{
var popup = $find('ModalPopupClientID');
popup.add_shown(SetFocus);
}
function SetFocus()
{
$get('TriggerClientId').focus();
}
i'm not sure tho if this will help you with calling it from the server side tho
add_shown()
just saved me a bunch of effort and worked perfectly!!
Awesome! I also had to add Sys.Application.add_load(pageLoad);
to my page to get it to fire the pageLoad()
Just to add to Jorin: I added my javascript via runtime, and the add_shown
would work when a partial postback was done (using an update panel) however, it would NOT when a full postback was done (due to dynamic controls triggering the MPE to be shown). Adding the Sys.Application.add_load(pageLoad);
line just before my javascript made it work even on full page postback.
You may also need some timeout in the shown handler, it seems some initialization in the popup extender itself takes place in a timeout. I needed that for some proper size calculations.
Here's a simple way to do it in markup:
<ajaxToolkit:ModalPopupExtender
ID="ModalPopupExtender2" runat="server"
TargetControlID="lnk_OpenGame"
PopupControlID="Panel1"
BehaviorID="SilverPracticeBehaviorID" >
<Animations>
<OnShown>
<ScriptAction Script="InitializeGame();" />
</OnShown>
</Animations>
</ajaxToolkit:ModalPopupExtender>
I get the following error: "Animation on TargetControlID="hiddenButtonPopup1"
uses property AjaxControlToolkit.ModalPopupExtender.OnShown
that does not exist or cannot be set". hiddenButtonPopup1
is a hidden button that is assigned to TargetControlID
.
Works great for me, and I like the markup-only solution seeing as how my JS is also in the markup
You should use the BehaviorID
value mpeBID
of your ModalPopupExtender
.
function pageLoad() {
$find('mpeBID').add_shown(HideMediaPlayer);
}
function HideMediaPlayer() {
var divMovie = $get('<%=divMovie.ClientID%>');
divMovie.style.display = "none";
}
If you are using a button or hyperlink or something to trigger the popup to show, could you also add an additional handler to the onClick
event of the trigger which should still fire the modal popup and run the javascript at the same time?
You can show the popup from client-side JS code, so without requiring a postback. I guess you could run additional JS code that way.
The ModalPopupExtender modifies the button/hyperlink that you tell it to be the "trigger" element. The onclick script I add triggers before the popup is shown. I want script to fire after the popup is shown.
Also, still leaves me with the problem of when I show the modal from server side.
TinyMCE work on invisible textbox if you hide it with css (display:none;
) You make an "onclick" event on TargetControlID, for init TinyMCE, if you use also an updatepanel
For two modal forms:
var launch = false;
var NameObject = '';
function launchModal(ModalPopupExtender) {
launch = true;
NameObject = ModalPopupExtender;
}
function pageLoad() {
if (launch) {
var ModalObject = $find(NameObject);
ModalObject.show();
ModalObject.add_shown(SetFocus);
}
}
function SetFocus() {
$get('TriggerClientId').focus();
}
Server side: behand
protected void btnNuevo_Click(object sender, EventArgs e)
{
//Para recuperar el formulario modal desde el lado del sercidor
ScriptManager.RegisterStartupScript(Page, Page.GetType(), "key", "<script>launchModal('" + ModalPopupExtender_Factura.ID.ToString() + "');</script>", false);
}
var launch = false;
function launchModal() {
launch = true;
}
function pageLoad() {
if (launch) {
var ModalPedimento = $find('ModalPopupExtender_Pedimento');
ModalPedimento.show();
ModalPedimento.add_shown(SetFocus);
}
}
function SetFocus() {
$get('TriggerClientId').focus();
}
How is this better than the previously accepted answer above?
Length of a JavaScript object I have a JavaScript object. Is there a built-in or accepted best practice way to get the length of this object? const myObject = new Object(); myObject["firstname"] = "Gareth"; myObject["lastname"] = "Simpson"; myObject["age"] = 21; that's kinda true, but so many people are used to PHP's "associative array" that they might assume it means "ordered associative map", when JS objects are in fact unordered. In the above example, myObject.length is undefined, at least in a browser environment. That's why it isn't valid Variants of Object.{keys, values, entries}(obj).length have now been mentioned a total of 38 times in 16 answers plus in the comments of this question, and another 11 times in 7 deleted answers. I think that’s enough now. 44 Answers Updated answer Here's an update as of 2016 and widespread deployment of ES5 and beyond. For IE9+ and all other…
How do I post and then redirect to an external URL from ASP_Net?
ASP_NET server-side controls postback to their own page. This makes cases where you want to redirect a user to an external page, but need to post to that page for some reason (for authentication, for instance) a pain. An HttpWebRequest works great if you don't want to redirect, and JavaScript is fine in some cases, but can get tricky if you really do need the server-side code to get the data together for the post. So how do you both post to an external URL and redirect the user to the result from your ASP_NET codebehind code? Here's how I solved this problem today. I started from this article on C# Corner, but found the example - while…
I'm working on a website that will switch to a new style on a set date. The site's built-in semantic HTML and CSS, so the change should just require a CSS reference change. I'm working with a designer who will need to be able to see how it's looking, as well as a client who will need to be able to review content updates in the current look as well as design progress on the new look. I'm planning to use a magic querystring value and/or a javascript link in the footer which…